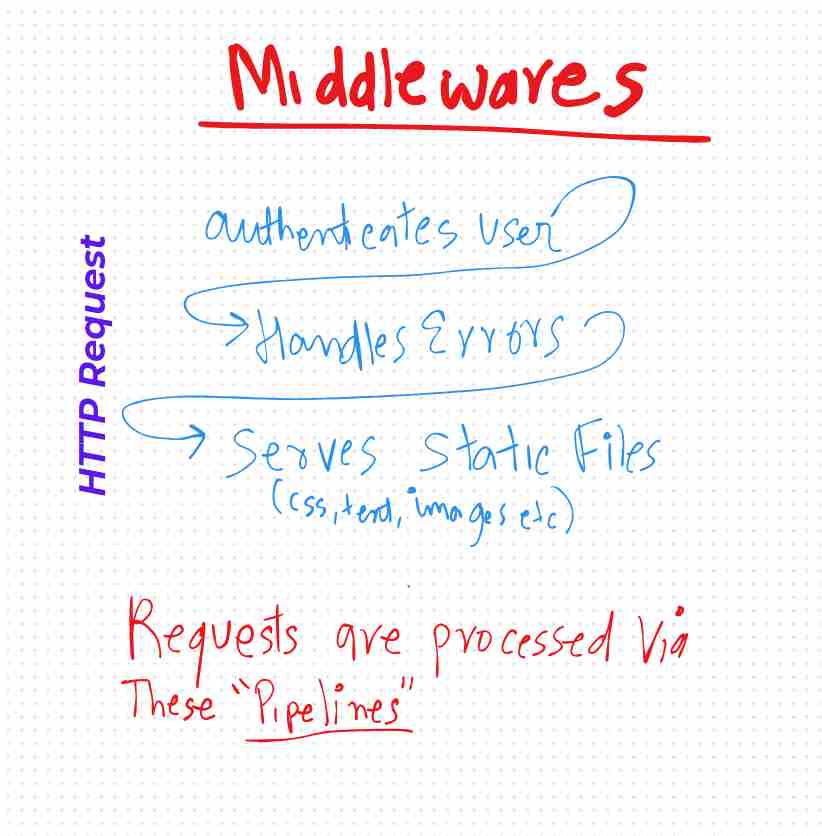
Middleware is a piece of software that handles http request and response.
In simple term, .net core works with middleware in order to enhance performance of our application rather than having big chunk of framework component/ libraries at once, for which most of the http requests, it’s not even useful.
Middleware is modular
Whenever request comes, it is forwarded to middleware, to perform some tasks related to request context. It then can pass request to do some further processing. .Net Core, we can have many middleware’s configured in pipeline, each one is responsible to perform related tasks like logging, authentication, serving static files, MVC etc. So, it follows the “single responsible principle” and provide modular way of configuring application. We can simply remove middleware which we do not required for some specific type of requests, in that way our application becomes so light.
“You only pay for the for the middleware components you have in your application request processing pipeline!”
- Every middleware has control – Incoming request, and outgoing response.
- Middleware component pass request to next middleware in the pipeline
Ex- one middleware does some processing, and then pass the request on to the next middleware for further processing.
- Middleware component may handle the request and short circuit the rest of the pipeline
Ex – if request is for serving static file, then no need to go to next pipeline, like MVC. It should stop. We can achieve that in .net core.
- Executed in the order they are added to the pipeline.
Other Advantages of Middleware
- Selective execution: With .NET Core middleware, you have the flexibility to choose and include only the middleware components you need for your application. This means you can avoid unnecessary processing and reduce the overall overhead. In contrast, the .NET Framework includes a comprehensive set of features, which may result in executing code that you don’t require for your specific application, potentially impacting performance.
- Asynchronous processing: .NET Core middleware is designed to take full advantage of asynchronous programming patterns, such as async/await. This allows for non-blocking I/O operations, enabling concurrent processing of multiple requests. Asynchronous processing improves the responsiveness and scalability of your application, especially under high load scenarios. The .NET Framework also supports asynchronous programming, but .NET Core provides better optimizations and tools for managing asynchronous tasks.
- Optimized request pipeline: The request pipeline in .NET Core middleware is designed for improved performance. It incorporates various optimizations, such as a lightweight execution model, efficient routing, and built-in features like response compression and caching. These optimizations help minimize latency and improve the overall speed of request processing.
- Improved platform compatibility: .NET Core middleware has been built with cross-platform compatibility in mind. It is designed to work seamlessly on Windows, Linux, and macOS, taking advantage of platform-specific optimizations. This flexibility allows you to choose the best hosting environment for your application and optimize performance based on the target platform.
- Enhanced development practices: .NET Core promotes modern development practices, such as dependency injection, which helps manage dependencies more efficiently and improves code maintainability. It also encourages the use of lightweight frameworks like ASP.NET Core, which provide better performance and enable you to build highly optimized web applications.
Configure Middleware
In Startup.cs there are two methods
- ConfigureServices”
The ConfigureServices method is responsible for configuring the services that your application will use. This includes registering dependencies, configuring dependency injection, and setting up various services needed by the application. Middleware-specific configurations are not typically done in this method, but it plays a crucial role in the overall configuration process.
- Configure:
The Configure method is where you configure the middleware pipeline for your application. This method defines the sequence of middleware components and their respective configurations. The middleware components are added using the app parameter of the Configure method, which is an instance of IApplicationBuilder.
Here’s an example of how to use the
Configure
method to add middleware components:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseCookiePolicy(); // Additional middleware configurations can be added here app.UseMvc(routes => { routes.MapRoute( name: "default", template: "{controller=Home}/{action=Index}/{id?}"); }); } |
In this example, several middleware components are added to the pipeline:
- UseDeveloperExceptionPage and UseExceptionHandler handle exceptions and provide developer-friendly error pages.
- UseHsts adds HTTP Strict Transport Security (HSTS) headers for enhanced security.
- UseHttpsRedirection redirects HTTP requests to HTTPS.
- UseStaticFiles serves static files like CSS, JavaScript, and images.
- UseCookiePolicy sets up cookie handling.
You can also add custom middleware components or third-party middleware by using the app.Use method and passing in the middleware component.
Remember to add the necessary using statements at the top of your Startup.cs file for the middleware components you want to use.
By configuring the middleware pipeline in the Configure method, you define the order in which middleware components are executed and the specific configurations applied to each middleware component.
How to add middleware components to the application’s request pipeline
1. app.Run Method:
The app.Run method is used to add a terminal middleware component to the request pipeline. It takes a RequestDelegate parameter that represents a delegate that handles the request. The delegate receives an instance of HttpContext and is responsible for generating the response.
Here’s an example of using app.Run to handle a request:
1 2 3 4 |
app.Run(async (context) => { await context.Response.WriteAsync("Hello, World!"); }); |
In this example, when a request comes in, the delegate defined in app.Run
will be executed, and the response “Hello, World!” will be sent back to the client.
2. app.Use Method:
The app.Use method is used to add middleware components to the request pipeline. Middleware components added with app.Use are non-terminal, meaning subsequent middleware components in the pipeline will also be executed.
Here’s an example of using
app.Use
to add a middleware component:
{
// Do something before the next middleware component
await next.Invoke();
// Do something after the next middleware component
});
In this example, the delegate passed to app.Use is responsible for processing the request and invoking the next middleware component in the pipeline by calling next.Invoke().
3. app.UseMiddleware: Method
The app.UseMiddleware method is another way to add middleware components to the request pipeline. It allows you to use custom middleware classes in your application.
Here’s an example of using app.UseMiddleware to add a custom middleware component:
In this example, CustomMiddleware is a custom middleware class that you have implemented. It should implement the IMiddleware interface or have a method with the signature Task InvokeAsync(HttpContext context, RequestDelegate next).
4. app.Map and app.MapWhen Methods:
The app.Map and app.MapWhen methods allow you to map specific request paths or conditions to different middleware branches. This enables you to apply different middleware components based on specific route patterns or conditions.
Here’s an example of using app.Map to map a specific path to a middleware branch:
1 2 3 4 5 6 |
app.Map("/admin", adminApp => { adminApp.UseMiddleware<adminmiddleware>(); }); </adminmiddleware> |
In this example, when a request comes in with a path starting with “/admin”, the AdminMiddleware will be executed.
These are some of the key methods used to configure middleware components in the request pipeline of a .NET Core application. By utilizing these methods, you can define the sequence of middleware components, handle specific routes or conditions, and customize the behavior of your application.
Thanks for reading, do not forget to put comments and suggestions
app.UseMiddleware
0 Comments