CLR
(Common Language Runtime)
Responsible for program execution in .Net Framework
Some crucial role of CLR-
Memory Management: The CLR is responsible for managing memory allocation and deallocation in .NET applications. It includes automatic memory management through garbage collection, which tracks and releases memory that is no longer in use, thus relieving developers from manual memory management.
Just-In-Time (JIT) Compilation: The CLR employs a JIT compiler to convert Intermediate Language (IL) code into machine code specific to the target platform during runtime. This process improves performance by optimizing code execution and adapting it to the underlying hardware.
Exception Handling: The CLR provides a robust exception handling mechanism. It allows developers to catch and handle exceptions, ensuring that applications can gracefully handle unexpected errors or exceptional situations during runtime.
Security and Code Access: The CLR enforces security measures to protect against unauthorized or malicious code execution. It implements code access security, which restricts code from performing actions that are not permitted based on defined permissions and trust levels. It helps maintain application integrity and prevents unauthorized access to sensitive resources.
“Here, Security means, code scope will be at our application level only, it will not interfere OS level security. Like File operations, changing the base OS files to make system venerable and so on.”
Language Interoperability: The CLR supports multiple programming languages, enabling them to interoperate seamlessly within the .NET ecosystem. It provides a common execution environment, runtime services, and a set of standard libraries that can be accessed by various .NET languages, promoting language interoperability and code reuse.
These are some of the crucial tasks performed by the CLR to ensure efficient and secure execution of .NET applications. The CLR’s capabilities enable developers to focus on writing code while benefiting from automatic memory management, performance optimizations, exception handling, and language interoperability provided by the runtime.
CLS
(Common language specification)
Means– Does not matter which programming language you wrote the code, by time of execution, all the language will convert to common language.
(MSIL) : (Microsoft Intermediate Language)
Also known as Common Intermediate Language. is a low-level, platform-independent bytecode language used by the .NET Framework.
MSIL is a platform-independent representation of the code.
Language designers have to use common subset of basic language feature that all language have to follow.
All will turn into MSIL code in the end.
Example-
VB code-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Module MainModule Sub Main() Dim num1 As Integer = 5 Dim num2 As Integer = 10 Dim sum As Integer = num1 + num2 Console.WriteLine("Sum: " & sum) End Sub End Module |
C# Code-
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
using System; class MainClass { static void Main() { int num1 = 5; int num2 = 10; int sum = num1 + num2; Console.WriteLine("Sum: " + sum); } } |
Converted Common code(MSIL)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
.method private hidebysig static void Main() cil managed { .entrypoint .maxstack 2 .locals init ( [0] int32 num1, [1] int32 num2, [2] int32 sum) ldc.i4.5 stloc.0 ldc.i4.s 10 stloc.1 ldloc.0 ldloc.1 add stloc.2 ldstr "Sum: " ldloc.2 box int32 call string [mscorlib]System.String::Concat(object, object) call void [mscorlib]System.Console::WriteLine(string) ret } |
CTS
All about data types!
It defines how types are declared, used and managed.
Enable cross language integration, type safety, ensure high performance code execution.
Also provide object-oriented model.
Provides a Library that contains the primitive data types (basic or fundamental data types like string, float, double, int etc.)
Example-
VB
Dim a As integer
C#
Int a;
Compilation
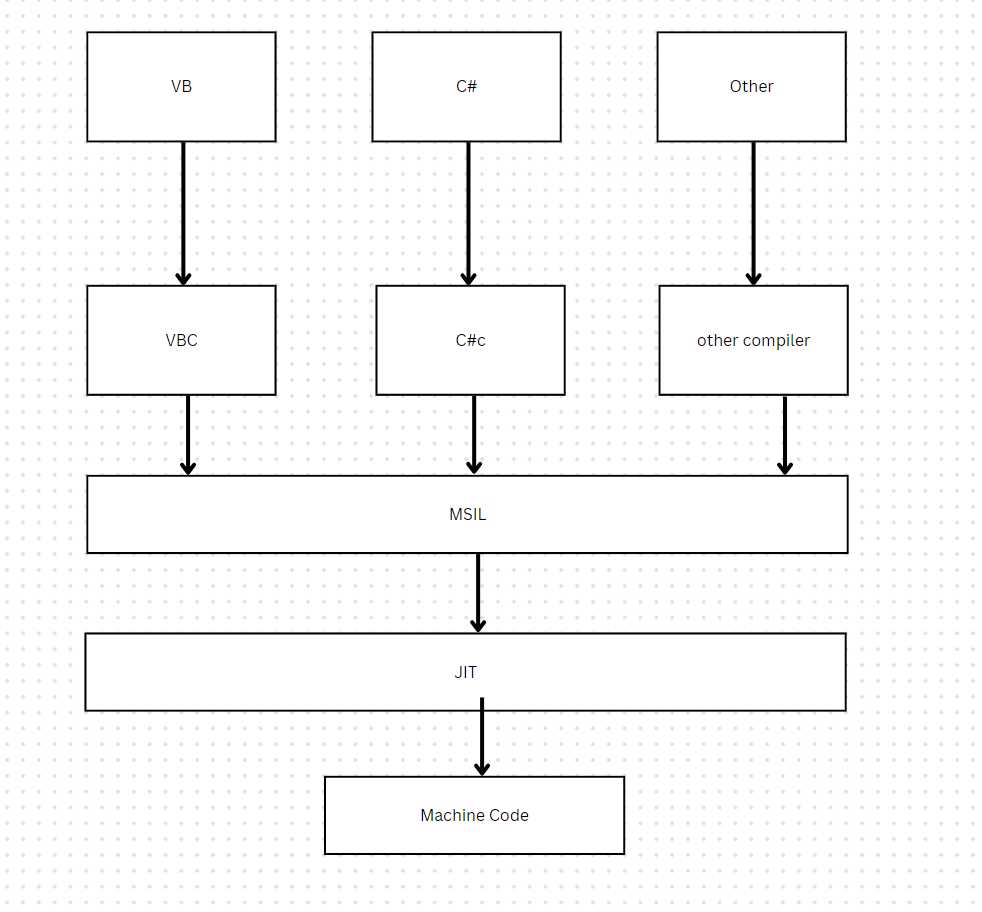
JIT:
Just in time compiler will not compile pile of code at once, like in application we have 50 webforms, but at a time, we are opening one form. So just in time compiler will compile the required code into Machine code on demand. Then enhance performance.
JIT target code is depended on Machines operating system like android, windows.
Hopefully I am able to explain this concept in easier way. In future I will improve this content, please comment below how I can make this easy to understand.
0 Comments